JAX-WS Call WS using Maven Use Maven to Generate all Stubs using 'wsimport' utility. After generating the webservice you can call the service using Java class. JAX-WS (JSR 224) API License: CDDL GPL 2.0: Categories: Java Specifications: Tags: standard javax xml webservice api specs: Used By: 720 artifacts.
There are many ways and techniques to create a web service client in java, however here, in my this blog I am creating a web service client project with JAX-WS using maven.
JAX-WS allows us to invoke a web service, as if we were making a local method call. For this to happen a standard mapping from WSDL to Java has been defined.
The Service Endpoint Interface (SEI) is the Java representation of the web service endpoint.
At runtime JAX-WS creates an instance of a SEI that can be used to make web service calls by simply making method calls on the SEI.
The stubs have been generated by the WSDL. So the stubs are a prerequisite.
Now to begin with, create a simple project using maven, this project would be your web service client.
1) Maven Configuration:
Into your pom.xml file add the following plugin:
<plugin>
<groupId>org.jvnet.jax-ws-commons</groupId>
<artifactId>jaxws-maven-plugin</artifactId>
<version>2.1</version>
<executions>
<execution>
<goals>
<goal>wsimport</goal>
</goals>
</execution>
</executions>
<configuration>
<packageName>com.sample.stub</packageName>
<wsdlDirectory>WebServiceDescription</wsdlDirectory>
<sourceDestDir>src/main/java</sourceDestDir>
</configuration>
</plugin>
Create a directory named WebServiceDescription, as specified in the above plugin (between the tag <wsdlDirectory>) and place your wsdl file into this directory.
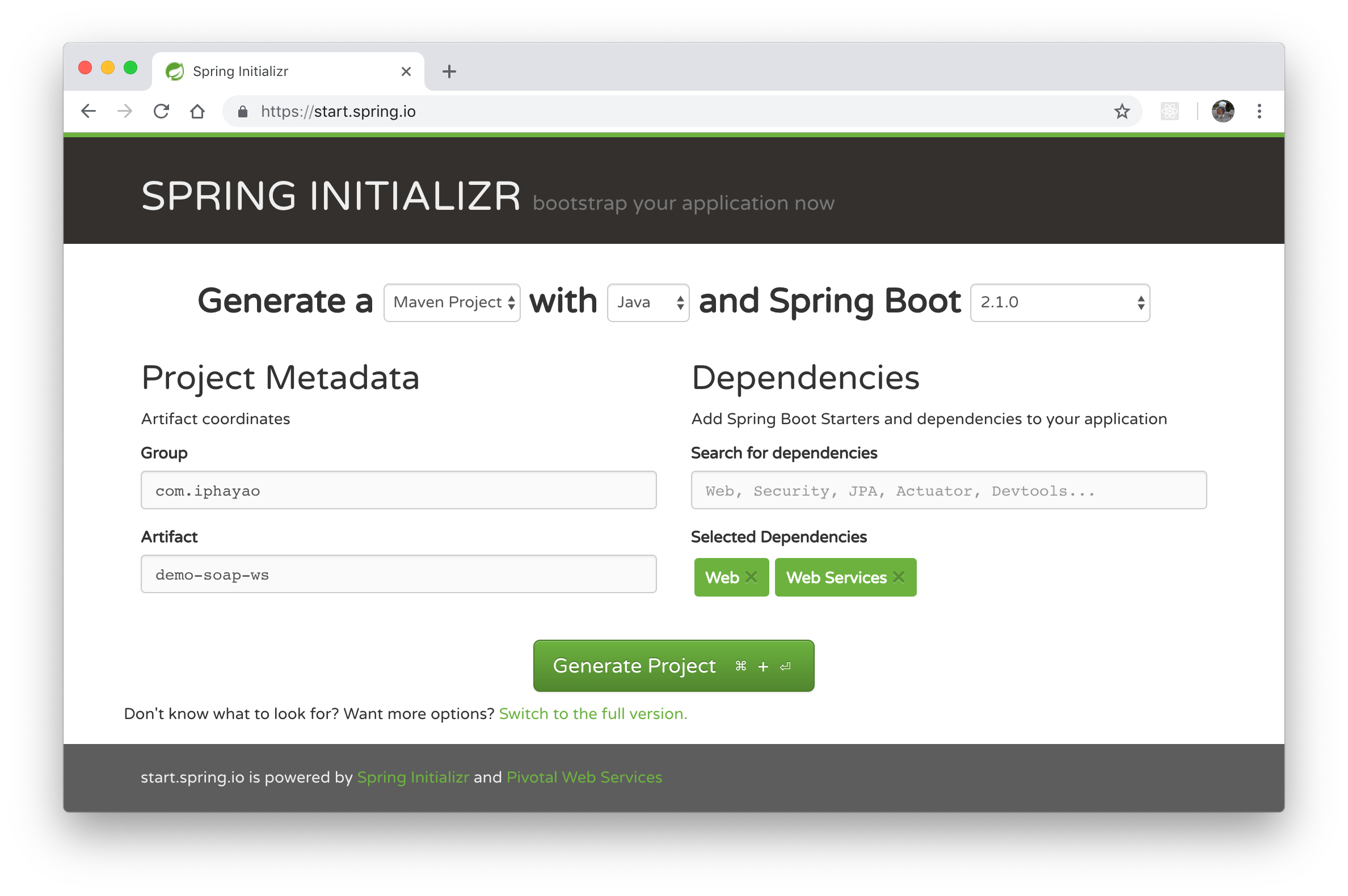
2) Generating stub classes:
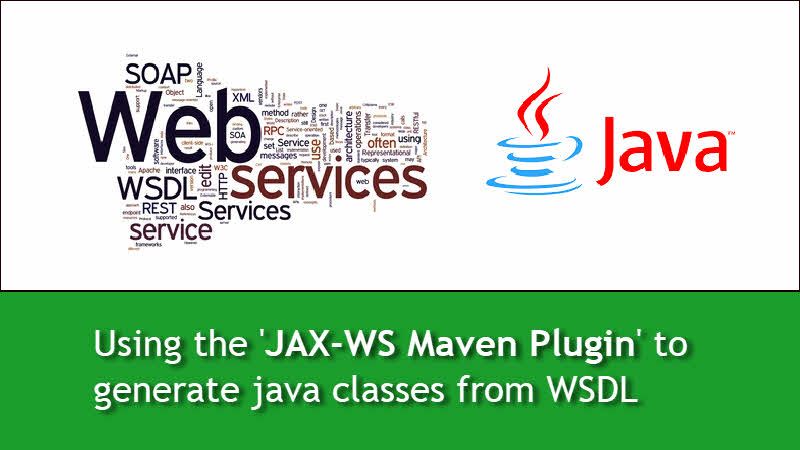
For generating stub use the following maven command
mvn clean jaxws:wsimport
The above command jaxws:wsimportgenerates JAX-WS portable artifacts used in JAX-WS clients and services. The tool reads a WSDL and generates all the required artifacts for web service development, deployment, and invocation.
Check the com.sample.stub package it should contain all the required stub classes. Which comprise of
Service class
Object-Factory class
service-method class
returntype class
package-info class etc.
3) Access the Web service:
Here is a custom Java class code used to access
System.out.println('Customer Name: '+response.getCustomerName());
e.printStackTrace();
}
- Now, let me explain some of the above code snippet.Beginning with QName, A
QName
object is an object that represents an XML qualified name. The object is composed of a namespace URI and the local part of the qualified name i.e. the service name CustomerInfoBeanService. - In the next line, the URL object file points to the WSDL that was deployed:
- http://localhost:7001/CustomerInfoBean/CustomerInfoBeanService?WSDL
- With the next line of code we are able to get through the service. The wsClient is the service object.
- Further we create a port, this can be done by invoking the get port method on service object. The method name usually begin is getPortTypePort() where PortType is as specified in wsdl.
- At this point we have got the service port. We can now invoke our method on this port.
Done, any comments are appreciated.
jaxws:wsimport-test
Full name:
org.codehaus.mojo:jaxws-maven-plugin:2.6:wsimport-test
Description:
Parses wsdl and binding files and generates Java code needed toaccess it (for tests).${maven.test.skip} property is honored. If it isset, code generation is skipped.
Attributes:
- Requires a Maven project to be executed.
- Requires dependency resolution of artifacts in scope: test.
- Binds by default to the lifecycle phase: generate-test-sources.
Optional Parameters
Name | Type | Since | Description |
---|---|---|---|
<args> | List | - | Specify optional command-line options. Multiple elements can be specified, and each token must beplaced in its own list. |
<bindingDirectory> | File | - | Directory containing binding files. Default value is: ${project.basedir}/src/jaxws. |
<bindingFiles> | List | - | List of files to use for bindings. If not specified, all.xml files in the bindingDirectory willbe used. |
<catalog> | File | - | Catalog file to resolve external entity references support TR9401,XCatalog, and OASIS XML Catalog format. |
<destDir> | File | - | Specify where to place output generated classes. Usexnocompile to turn this off. Default value is: ${project.build.testOutputDirectory}. |
<encoding> | String | - | Specify character encoding used by source files. Default value is: ${project.build.sourceEncoding}. |
<executable> | File | 2.2.1 | Path to the executable. Should be either wsgen orwsimport but basically any script which willunderstand passed in arguments will work. |
<extension> | boolean | - | Allow to use the JAXWS Vendor Extensions. Default value is: false. |
<genJWS> | boolean | - | Generate stubbed JWS implementation file. Default value is: false. |
<httpproxy> | String | - | Set HTTP/HTTPS proxy. Format is[user[:password]@]proxyHost[:proxyPort]. |
<implDestDir> | File | - | Specify where to generate JWS implementation file. Default value is: ${project.build.testSourceDirectory}. |
<implPortName> | String | - | Local portion of port name for generated JWS implementation.Implies genJWS=true. Note: It is a QName string,formatted as: '{' + Namespace URI + '}' + local part |
<implServiceName> | String | - | Local portion of service name for generated JWS implementation.Implies genJWS=true. Note: It is a QName string,formatted as: '{' + Namespace URI + '}' + local part |
<keep> | boolean | - | Keep generated files. Default value is: true. |
<packageName> | String | - | The package in which the source files will be generated. |
<quiet> | boolean | - | Suppress wsimport output. Default value is: false. |
<skip> | boolean | - | Set this to 'true' to bypass code generation. User property is: maven.test.skip. |
<sourceDestDir> | File | - | Specify where to place generated source files, keep is turned onwith this option. Default value is: ${project.build.directory}/generated-sources/test-wsimport. |
<staleFile> | File | - | The folder containing flag files used to determine if the output isstale. Default value is: ${project.build.directory}/jaxws/stale. |
<target> | String | - | Generate code as per the given JAXWS specification version. Setting'2.0' will cause JAX-WS to generate artifacts that run with JAX-WS2.0 runtime. |
<useJdkToolchainExecutable> | boolean | 2.4 | If a JDK toolchain is found, by default, it is used to getjava executable with its tools.jar. Butif set to true, it is used it to findwsgen and wsimport executables. Default value is: false. |
<verbose> | boolean | - | Output messages about what the tool is doing. Default value is: false. |
<vmArgs> | List | - | Specify optional JVM options. Multiple elements can be specified, and each token must beplaced in its own list. |
<wsdlDirectory> | File | - | Directory containing WSDL files. Default value is: ${project.basedir}/src/wsdl. |
<wsdlFiles> | List | - | List of files to use for WSDLs. If not specified, all.wsdl files in the wsdlDirectory will beused. |
<wsdlLocation> | String | - | @WebService.wsdlLocation and @WebServiceClient.wsdlLocation value. Can end with asterisk in which case relative path of the WSDLwill be appended to the given wsdlLocation. Example: wsdlLocation for a.wsdl will behttp://example.com/mywebservices/a.wsdlwsdlLocation for b/b.wsdl will behttp://example.com/mywebservices/b/b.wsdl wsdlLocation for ${project.basedir}/src/mywsdls/c.wsdlwill be file://absolute/path/to/c.wsdl Note: External binding files cannot be used if asterisk notationis in place. |
<wsdlUrls> | List | - | List of external WSDL URLs to be compiled. |
<xadditionalHeaders> | boolean | - | Maps headers not bound to the request or response messages to Javamethod parameters. Default value is: false. |
<xauthFile> | File | - | Specify the location of authorization file. |
<xdebug> | boolean | - | Turn on debug message. Default value is: false. |
<xdisableAuthenticator> | boolean | - | Disable Authenticator used by JAX-WS RI, xauthfilewill be ignored if set. Default value is: false. |
<xdisableSSLHostnameVerification> | boolean | - | Disable the SSL Hostname verification while fetching WSDL(s). Default value is: false. |
<xjcArgs> | List | - | Specify optional XJC-specific parameters that should simply bepassed to xjc using -B option of WsImportcommand. Multiple elements can be specified, and each token must beplaced in its own list. Star jalsha serial time 2019. |
<xnoAddressingDataBinding> | boolean | - | Binding W3C EndpointReferenceType to Java. By default WsImportfollows spec and does not bind EndpointReferenceType to Java anduses the spec provided W3CEndpointReference Default value is: false. |
<xnocompile> | boolean | - | Turn off compilation after code generation and let generatedsources be compiled by maven during compilation phase; keep isturned on with this option. Default value is: true. |
<xuseBaseResourceAndURLToLoadWSDL> | boolean | - | If set, the generated Service classes will load the WSDL file froma URL generated from the base resource. Default value is: false. |
Parameter Details
<args>
Specify optional command-line options.Multiple elements can be specified, and each token must beplaced in its own list.
- Type: java.util.List
- Required: No
<bindingDirectory>
- Type: java.io.File
- Required: No
- Default: ${project.basedir}/src/jaxws
<bindingFiles>
- Type: java.util.List
- Required: No
<catalog>
- Type: java.io.File
- Required: No
<destDir>
- Type: java.io.File
- Required: No
- Default: ${project.build.testOutputDirectory}
<encoding>
- Type: java.lang.String
- Required: No
- Default: ${project.build.sourceEncoding}
<executable>
- Type: java.io.File
- Since: 2.2.1
- Required: No
<extension>
- Type: boolean
- Required: No
- Default: false
<genJWS>
- Type: boolean
- Required: No
- Default: false
<httpproxy>
- Type: java.lang.String
- Required: No
<implDestDir>
- Type: java.io.File
- Required: No
- Default: ${project.build.testSourceDirectory}
<implPortName>
- Type: java.lang.String
- Required: No
<implServiceName>
- Type: java.lang.String
- Required: No
<keep>
- Type: boolean
- Required: No
- Default: true
<packageName>
- Type: java.lang.String
- Required: No
<quiet>
- Type: boolean
- Required: No
- Default: false
<skip>
- Type: boolean
- Required: No
- User Property: maven.test.skip
<sourceDestDir>
- Type: java.io.File
- Required: No
- Default: ${project.build.directory}/generated-sources/test-wsimport
<staleFile>
- Type: java.io.File
- Required: No
- Default: ${project.build.directory}/jaxws/stale
<target>
- Type: java.lang.String
- Required: No
<useJdkToolchainExecutable>
- Type: boolean
- Since: 2.4
- Required: No
- Default: false
<verbose>
- Type: boolean
- Required: No
- Default: false
<vmArgs>
Specify optional JVM options.Multiple elements can be specified, and each token must beplaced in its own list.
- Type: java.util.List
- Required: No
<wsdlDirectory>
- Type: java.io.File
- Required: No
- Default: ${project.basedir}/src/wsdl
<wsdlFiles>
- Type: java.util.List
- Required: No
<wsdlLocation>
@WebService.wsdlLocation and @WebServiceClient.wsdlLocation value.Can end with asterisk in which case relative path of the WSDLwill be appended to the given wsdlLocation.
Example:
wsdlLocation for a.wsdl will behttp://example.com/mywebservices/a.wsdlwsdlLocation for b/b.wsdl will behttp://example.com/mywebservices/b/b.wsdl
wsdlLocation for ${project.basedir}/src/mywsdls/c.wsdlwill be file://absolute/path/to/c.wsdl
Note: External binding files cannot be used if asterisk notationis in place.
- Type: java.lang.String
- Required: No
<wsdlUrls>
- Type: java.util.List
- Required: No
<xadditionalHeaders>
- Type: boolean
- Required: No
- Default: false
<xauthFile>
- Type: java.io.File
- Required: No
<xdebug>
- Type: boolean
- Required: No
- Default: false
<xdisableAuthenticator>
- Type: boolean
- Required: No
- Default: false
<xdisableSSLHostnameVerification>
- Type: boolean
- Required: No
- Default: false
<xjcArgs>
Specify optional XJC-specific parameters that should simply bepassed to xjc using -B option of WsImportcommand.Multiple elements can be specified, and each token must beplaced in its own list.
- Type: java.util.List
- Required: No
<xnoAddressingDataBinding>
- Type: boolean
- Required: No
- Default: false
<xnocompile>
- Type: boolean
- Required: No
- Default: true
<xuseBaseResourceAndURLToLoadWSDL>
- Type: boolean
- Required: No
- Default: false